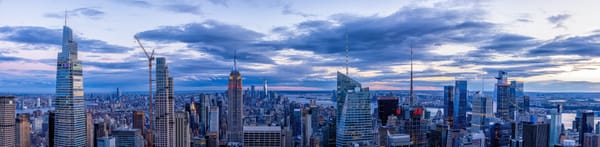
Photography
Skyscraper Symphony – A Sunset Panorama of Midtown Manhattan
A breathtaking panoramic view of Midtown Manhattan at sunset — from the iconic Empire State Building to the modern marvels of Hudson Yards, this photo captures the quiet majesty of New York City’s skyline in a rare moment of stillness.